Understanding Scala: A Modern and Powerful Language for the JVM
January 20, 2025 • 5 min read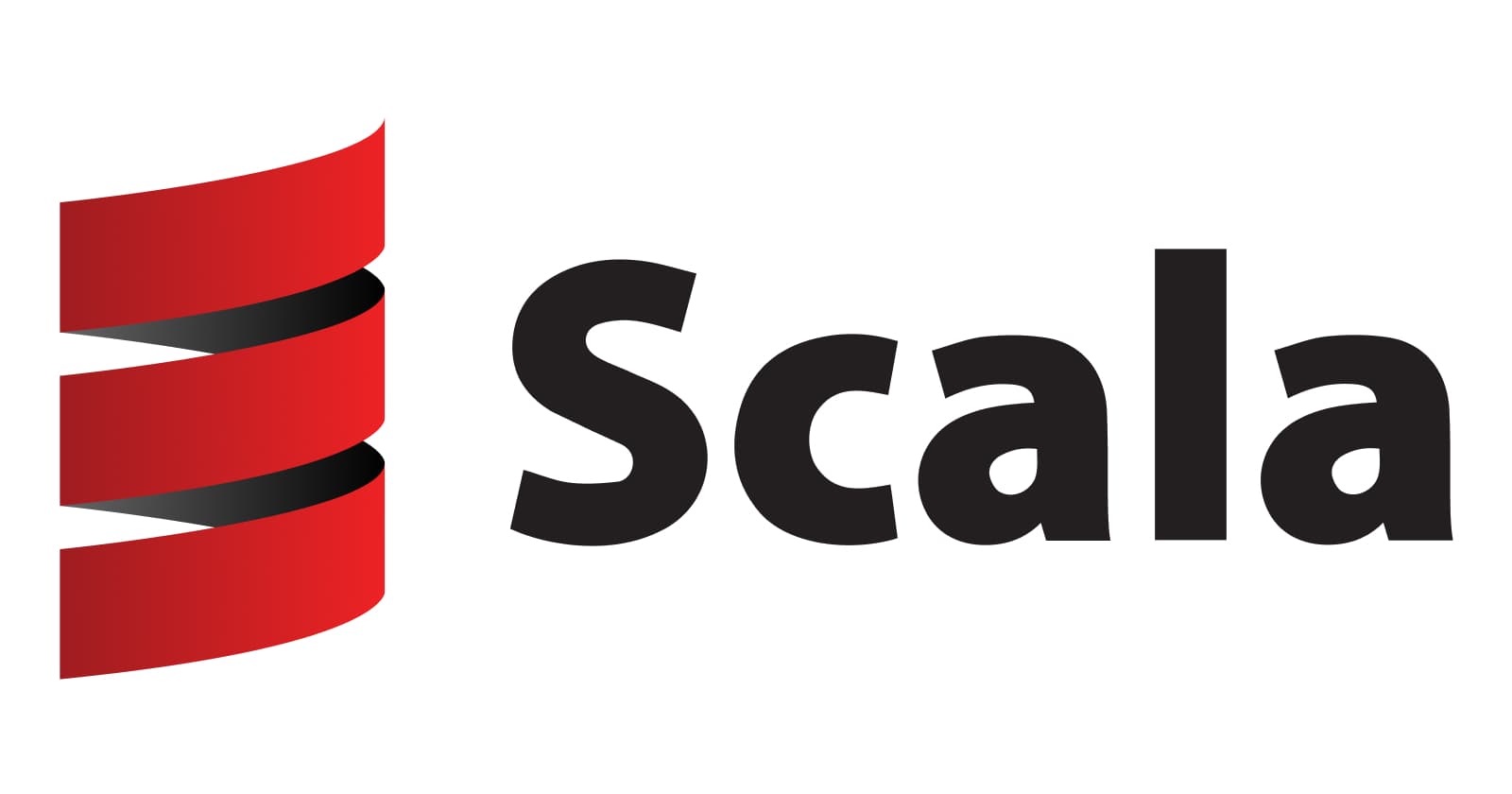
Scala is a multi-paradigm programming language that combines the best features of both object-oriented and functional programming. It is a powerful language that runs on the Java Virtual Machine (JVM) and is interoperable with Java. In this article, we will explore why Scala is gaining popularity among developers, how it blends functional and object-oriented paradigms, and why it might be a good choice for your next project.
What is Scala?
Scala, short for scalable language, was created by Martin Odersky in 2003 and officially released in 2004. The language was designed to address the limitations of Java while offering a more concise and expressive syntax. Scala runs on the JVM, which means it is fully compatible with Java libraries, and it also supports features such as pattern matching, higher-order functions, and immutability, making it more modern and expressive than Java.
Key Features of Scala
1. Object-Oriented and Functional Programming
Scala seamlessly integrates both object-oriented and functional programming principles. Every value in Scala is an object, and every function is a method. You can use classes, objects, and traits for object-oriented design and higher-order functions, immutability, and pattern matching for functional programming.
2. Concise Syntax
One of the standout features of Scala is its concise and expressive syntax. Scala code is often more compact compared to Java, reducing boilerplate code. For example, defining a simple function in Scala can be done in just a few lines, compared to the verbose syntax of Java.
// Scala function example
def add(a: Int, b: Int): Int = a + b
In contrast, the same function in Java would require a class and explicit return statements.
// Java function example
public class Main {
public static int add(int a, int b) {
return a + b;
}
}
3. Immutability and Functional Programming
Scala encourages immutability, a core concept in functional programming. Immutable data structures ensure that once a value is assigned, it cannot be modified, which can lead to fewer bugs and better maintainability in concurrent or parallel programming scenarios.
val numbers = List(1, 2, 3) // The list is immutable, so we can't change its elements
4. Type Inference
Scala has a sophisticated type inference system, which allows developers to write cleaner code without explicitly specifying types for every variable. The Scala compiler can infer the types based on the code, making the language both flexible and safe.
val x = 42 // Scala infers that x is of type Int
5. Pattern Matching
Pattern matching is one of the most powerful features in Scala. It allows you to match data structures against patterns, making it easy to extract values and handle different cases in a more readable and maintainable way.
def describe(x: Any): String = x match {
case 1 => "One"
case "hello" => "Greeting"
case _ => "Unknown"
}
In this example, the describe
function matches different types and values, simplifying conditional logic.
6. Immutable Collections
Scala’s standard library includes a variety of immutable collections, such as List
, Set
, and Map
, which prevent side effects and make functional programming easier. Immutable collections are ideal for multithreaded environments since they are safe to share across threads without causing race conditions.
Scala vs. Java
While Scala runs on the JVM and is interoperable with Java, it offers several advantages over Java:
Conciseness: Scala is more concise than Java, which makes the code easier to read and write. This is especially beneficial when dealing with large codebases.
Immutability: Scala promotes immutability by default, which helps prevent side effects and makes code more predictable.
Higher-Order Functions: Scala supports first-class functions and higher-order functions, enabling more flexible and reusable code.
Advanced Features: Scala supports advanced features such as pattern matching, traits, and type classes, which are not available in Java.
Concurrency and Parallelism: With Scala’s functional programming capabilities, writing concurrent and parallel code is easier and less error-prone compared to Java’s traditional multi-threading approach.
However, Java still holds its ground in many enterprise environments due to its stability, vast ecosystem, and widespread adoption.
Popular Frameworks and Libraries in Scala
Scala has a number of powerful frameworks and libraries that make it even more appealing to developers:
Akka: A toolkit and runtime for building highly concurrent, distributed, and resilient message-driven applications.
Play Framework: A web application framework that allows developers to build scalable and reactive web applications.
Spark: A fast and general-purpose cluster computing system for big data processing. Apache Spark is written in Scala and is one of the most popular big data frameworks in the world.
Slick: A functional-relational mapping (FRM) library for working with databases in a Scala-centric way.
When to Use Scala
Scala is a great choice for a variety of applications, especially in the following scenarios:
Big Data: Scala is widely used in big data processing with Apache Spark, making it a go-to language for data scientists and engineers working with large datasets.
Concurrent and Distributed Systems: The Akka framework makes Scala an excellent choice for building highly concurrent and distributed systems.
Web Development: With frameworks like Play, Scala can be used to build reactive web applications that handle high traffic loads efficiently.
Functional Programming Enthusiasts: If you are a functional programming enthusiast, Scala offers a modern, JVM-compatible approach to functional programming with many powerful tools and features.
Conclusion
Scala is a versatile, high-performance language that offers both object-oriented and functional programming features. Its concise syntax, powerful functional programming capabilities, and strong interoperability with Java make it an attractive choice for developers. Whether you're building a large-scale distributed system, working with big data, or simply looking to improve your functional programming skills, Scala provides the tools and features to get the job done.
If you’re already familiar with Java, Scala offers an easy transition while providing access to more modern programming paradigms and features. As the demand for scalable, concurrent systems grows, Scala’s popularity is likely to continue increasing, making it a language worth learning for modern software development.