How to Create a Discord Bot
February 24, 2025 • 3 min read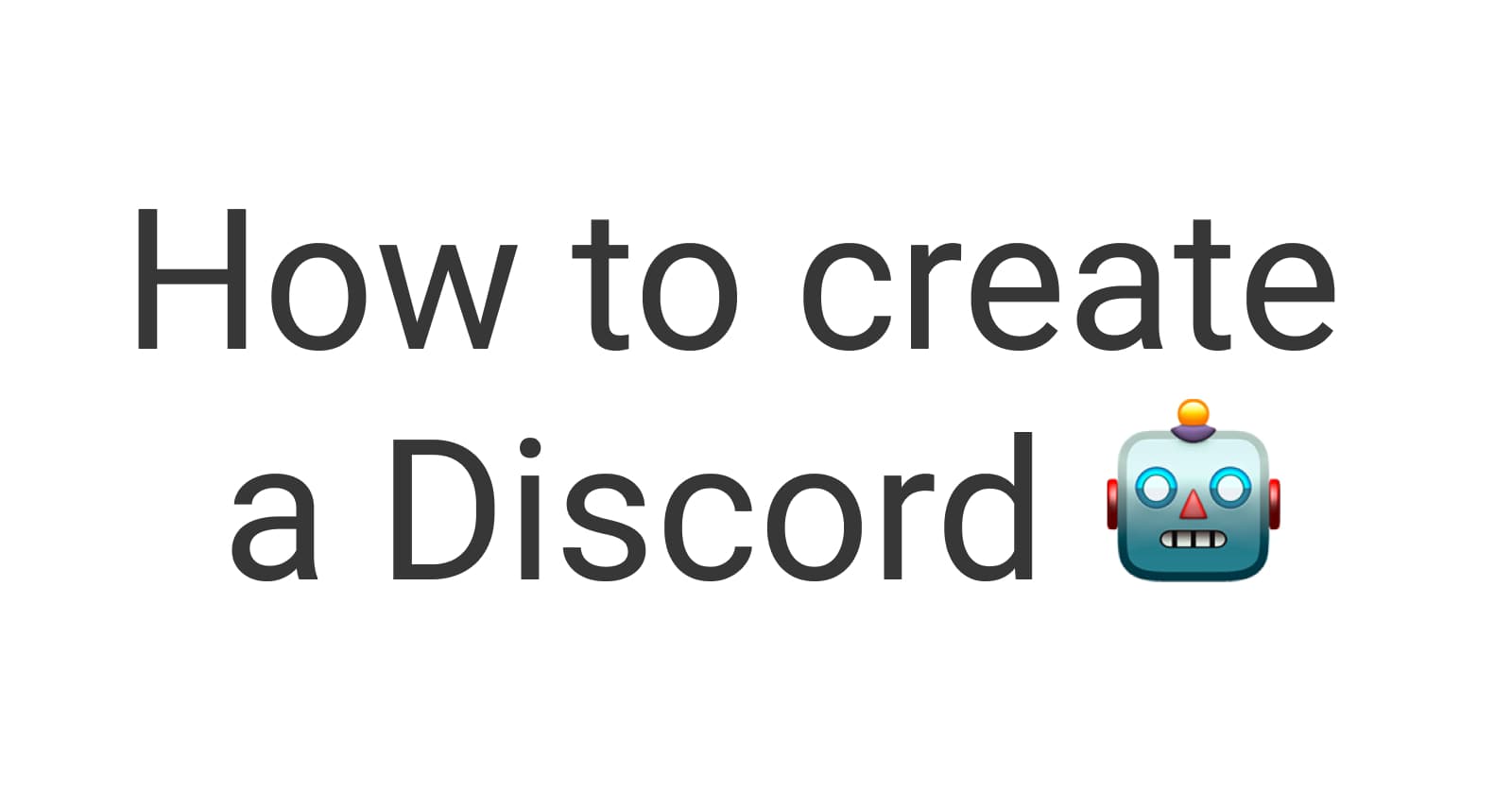
Discord bots are a great way to automate tasks, moderate servers, and enhance user engagement. Whether you want a bot for moderation, games, music, or other utilities, creating a bot is simpler than it may seem. This guide will walk you through the steps of building your own Discord bot using Node.js and Discord.js.
Prerequisites
Before we begin, ensure you have the following:
Node.js installed on your computer. You can download it from nodejs.org.
A Discord account and a server where you have admin privileges.
Basic JavaScript knowledge to understand the coding aspect.
Step 1: Create a New Discord Application
Go to the Discord Developer Portal.
Click on New Application and give your bot a name.
Navigate to the Bot tab on the left panel.
Click Add Bot, then customize its name and profile picture.
Under "Token", click "Reset Token" and copy it. This is important as it allows your bot to authenticate itself.
Step 2: Set Up Your Project
Open a terminal and create a new folder for your bot project:
mkdir discord-bot cd discord-bot
Initialize a new Node.js project:
npm init -y
Install the required dependencies:
npm install discord.js dotenv
Step 3: Create the Bot Script
Create a new file named
index.js
in your project folder.Open the file in a code editor (e.g., VS Code) and paste the following code:
require('dotenv').config(); const { Client, GatewayIntentBits } = require('discord.js'); const client = new Client({ intents: [GatewayIntentBits.Guilds, GatewayIntentBits.GuildMessages, GatewayIntentBits.MessageContent] }); client.once('ready', () => { console.log(`Logged in as ${client.user.tag}!`); }); client.on('messageCreate', message => { if (message.content === '!hello') { message.channel.send('Hello, world!'); } }); client.login(process.env.TOKEN);
Step 4: Store Your Token Securely
Create a
.env
file in the root folder and add:TOKEN=your-bot-token-here
Update your
package.json
to include:"scripts": { "start": "node index.js" }
Step 5: Invite Your Bot to a Server
Go back to the Discord Developer Portal.
Navigate to the OAuth2 tab and select URL Generator.
Choose bot as a scope and select necessary permissions (e.g.,
Send Messages
).Copy the generated link and paste it into your browser to invite the bot to your server.
Step 6: Run Your Bot
In the terminal, start your bot with:
npm start
You should see Logged in as [bot name]!
in the console, meaning your bot is live.
Conclusion
You have successfully created a basic Discord bot! You can expand it by adding more commands, integrating APIs, or even making it a fully functional assistant. Explore the Discord.js documentation for more advanced features.